Many businesses have invested thousands of man-hours into ASP.NET Web Forms. These same businesses don't have time to rewrite their systems using the latest Web technologies, such as MVC. However, with just a little reworking of your Web Forms, you can still get all the advantages of modern Web techniques, such as HTML 5, CSS 3, Bootstrap, and jQuery. Lately, I've been assisting many clients as they upgrade their Web Forms applications to take advantage of Bootstrap, jQuery, JavaScript, friendly URLs, and many other modern Web application techniques.
This article is another in my series on how to upgrade your Web Forms application without rewriting. In this article, I'll present how you can take advantage of HTML 5 generation from Text Box controls, use the new HTML 5 attributes and input types, and see techniques for working with jQuery.
HTML 5 and Visual Studio
Prior to HTML 5, you were limited to an input type of text for normal textual input by a user. To restrict input to numbers, dates, or any other domain type, you wrote JavaScript/jQuery. HTML 5 defines several new input types such as number
, date
, email
, etc. The browser now enforces the entry of the data matching the input type. This means that you'll write much less code! In addition, when a user data on a mobile phone, most of these types bring up the appropriate keyboard for the data entry.
Visual Studio 2010 had a service pack (Web Standards Update for Microsoft Visual Studio 2010 SP1) that you could download to add on the new HTML 5 input types to the TextBox control. Visual Studio 2012/2013 has the HTML 5 input types built right into the TextMode
property in IntelliSense and the Property Window (see Figure 1).
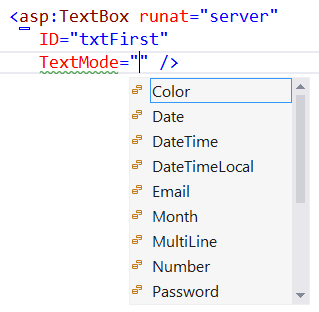
The newer browsers recognize all of the new HTML 5 input types. If they don't recognize one, the browser treats them as text. This means that you can start using these types immediately. If you have a JavaScript library that you use to enforce data types, most likely it will still work for the older browsers.
A better approach is to use the Modernizr() library to detect which features and input types your browser recognizes and only bring in your old JavaScript library if needed. When you create a Web Form application in Visual Studio 2012/2013, the Modernizr library is included automatically as a part of the project.
To use any of the new HTML 5 input types, you add a TextBox control to a Web page or go into an existing Web page and select the input type from the TextMode
property. All of the new HTML 5 input types are outlined in Table 1.
To use an ASP.NET TextBox control that requires a Date input type, your Web Form markup looks like the following code snippet:
<asp:TextBox runat="server"
TextMode="Date">
</asp:TextBox>
When this code generates, the resulting HTML looks like the following:
<input name="ctl02" type="date" />
The above code is valid HTML 5 and all modern browsers will recognize this as a date input type. Many of those browsers display a drop-down calendar control from which the user can select a date. If a browser doesn't recognize the date input type, it treats it as a text type.
Customizing Your Input Using Attributes
You can further customize the input types by applying attributes to your input fields. Even though these attributes don't display in the IntelliSense list of your ASP.NET TextBox controls, you can emit them in the final HTML by adding them to the TextBox control. There are several HTML 5 attributes that you can use, as shown in Table 2. An example of the autofocus and required attributes are shown in the following code snippet:
<asp:TextBox runat="server"
ID="txtFirst"
autofocus="autofocus"
required="required"
TextMode="SingleLine" />
Server and jQuery Controls
Prior to .NET 4, when ASP.NET controls generated HTML, the ID attribute that uniquely identified an HTML control was “munged” into a value that was not necessarily what you set it to in your server control. For example, if you use a TextBox control and set the name to txtProductName
, the resulting ID in the generated HTML might be MainContent_txtProductName
. When you're using all server-side code, this isn't a problem. However, once you start to use JavaScript and jQuery, you now have to know what the client-side ID attribute will be.
A work-around that we've employed for years in Web Forms is to use some script code with the ClientID
property to set the generated ID within some JavaScript code. The following code snippet shows an example of setting the value of your txtProductName
text box using a jQuery selector with the script code to give you the generated client-side ID.
<script>
function SetProductName() {
$("#<%=txtProductName.ClientID %>")
.val("A New Product");
}
</script>
Most of us know what we're doing and can ensure that we won't generate duplicate IDs within an HTML page, so it would be nice if we didn't have to work with these “munged” IDs that Microsoft generates. You now have that option by using the ClientIDMode
property. This property can be set in the Web.Config, in a Master Page, in a Page, or even on an individual control. There are four different values you may set this property to, as shown in Table 3.
If you wish to simplify your JavaScript/jQuery programming, set the ClientIDMode
to Static
. When you use Static
, you know that the ID you create on your server control is the same on the client side. Thus, you may use code like the following on your client side.
<script>
function SetProductName() {
$("#txtProductName").val("A New Product");
}
</script>
One thing you need to be aware of when using the Static
mode is that you may hit problems when working with user controls. If you use the same user control multiple times on the same page, you could end up with duplicate IDs. In this case, you might want to set the user control to continue to use ClientIDMode=“Auto”. By the way, you'll have the same issue when you use multiple partial views on the same page in MVC. You'll also have to add some prefix to your controls within the partial view in order to not duplicate the ID.
Where to Set ClientIDMode
You have a few options on where to set the ClientIDMode
. First, you can set it in the Web.Config if you wish to have every page use the same mode. Within the <pages>
element, add the clientIDMode="Static"
in order to make all pages use the static mode of ID generation.
<system.web>
<pages clientIDMode="Static" />
</system.web>
You may also set the ClientIDMode
within a master page in your site. Setting ClientIDMode="Static"
within the master page makes all pages that use that master page generate static IDs.
<%@ Master Language="C#"
ClientIDMode="Static"
Inherits="Samples.Site" %>
You may also set the ClientIDMode
on an individual Web page in the @Page
directive. Or, you can set the ClientIDMode
even on an individual control. As in most things in the Web world, the closest setting to a control wins. So even though you set the Web.Config setting of ClientIDMode
equal to Static
, if you set a TextBox control on an individual page to AutoID
, then AutoID
is what is used because it is the closest setting to the control.
Summary
Microsoft is actively adding new features and updating the underlying ASP.NET engine to support both Web Forms and MVC. In Visual Studio 2013, a new Web Forms project uses the same JavaScript and jQuery libraries, Bootstrap, Modernizr, and other technologies that are also included in a new MVC project. The existing server controls in Web Forms can take full advantage of HTML 5 and CSS 3. With the use of the new ClientIDMode
directive, you can now generate JavaScript/jQuery friendly control IDs as well.
Table 1: There are many HTML 5 input types available to use in your Web applications.
Property | Description |
SingleLine | Same as text and is the default type for any text box |
MultiLine | Same as text but allows multiple lines of text. |
Password | The input is text, but as a user types, the characters are overwritten with a blocked out character. |
Color | A color picker that returns a hex value for the color. |
Date | A date picker that returns a short date string. |
DateTime | A date and time picker that returns a long date string. |
DateTimeLocal | A date and time picker that returns a long date string in the browser's local format. |
A validated email address. | |
Month | A month picker that returns a month name and year. |
Number | A validated numeric value. |
Range | A range picker, usually depicted as a slider and returns a numeric value. |
Search | A text field with an x displayed for clearing the field. |
Phone | A text field. No validation is performed, but mobile phones will generally display the phone keyboard. |
Url | A validated Internet URL address. |
Week | A week picker that returns the week number of the year. |
Table 2: You can customize your input fields using HTML 5 attributes.
Attribute | Description |
autocomplete | Can be set to on or off. Determines whether or not a browser can fill in values that a user has entered before. |
autofocus | Set on one specific input field to place the cursor into this field when the page is displayed. |
list | A pre-defined list of values to display in a drop-down below a text box. Allows the user to type in a value or select from the list. |
min and max | Controls the minimum and maximum values for number, range, date, month, time, and week. |
multiple | When present, allows the user to enter more than one value for the email and file input types. |
pattern | A regular expression used to check the users input against. |
placeholder | A hint displayed in an input field when the field does not have focus. |
required | Whether or not an input field must contain a value. |
step | Used to specify valid intervals for an input such as number, range, date, month, time, and week. |
Table 3: Choose the ClientIDMode value based on the needs of your application.
Setting | Description |
AutoID | Generates an ID using any parent container followed by the ID you set on the control. For example "ParentControlName_IDName". |
Static | Uses the ID you set on the control. |
Predictable | Use this when you have data-bound controls (a GridView, DataList, etc.) and you need a unique ID number for each row generated from the control. |
Inherit | Use the ClientIDMode set by the parent control's ClientIDMode setting. |