How to structure a web application with the MVC pattern
Two patterns for servlet/JSP applications
A pattern is a standard approach that programmers use to solve common programming problems. This topic describes two patterns that you can use when you develop Java web applications. However, for a serious web application, most developers consider the second pattern to be a best practice and recommend avoiding the first pattern.
The Model 1 pattern
The Model 1 pattern that’s shown in Figure 2-1 uses a JSP to handle both the request and response of the application. In addition, the JSP does all of the processing for the application.
To do that, the JSP interacts with Java classes and objects that represent the data of the business objects in the application and provide the methods that do the business processing. In this figure, for example, the application stores the data for a user in a User object.
To save the data of the business classes, the application maps the data to a database or files that can be called the data store for the application. This is also known as persistent data storage because it exists after the application ends. Usually, data access classes like the UserDB class shown in this figure store the data of the business objects in a database. Later in this book, you’ll learn two ways to create data access classes like this one.
Although the Model 1 pattern is sometimes adequate for applications that have limited processing requirements, this pattern is not recommended for most applications. As a result, it isn’t presented in this book.
The Model 1 pattern
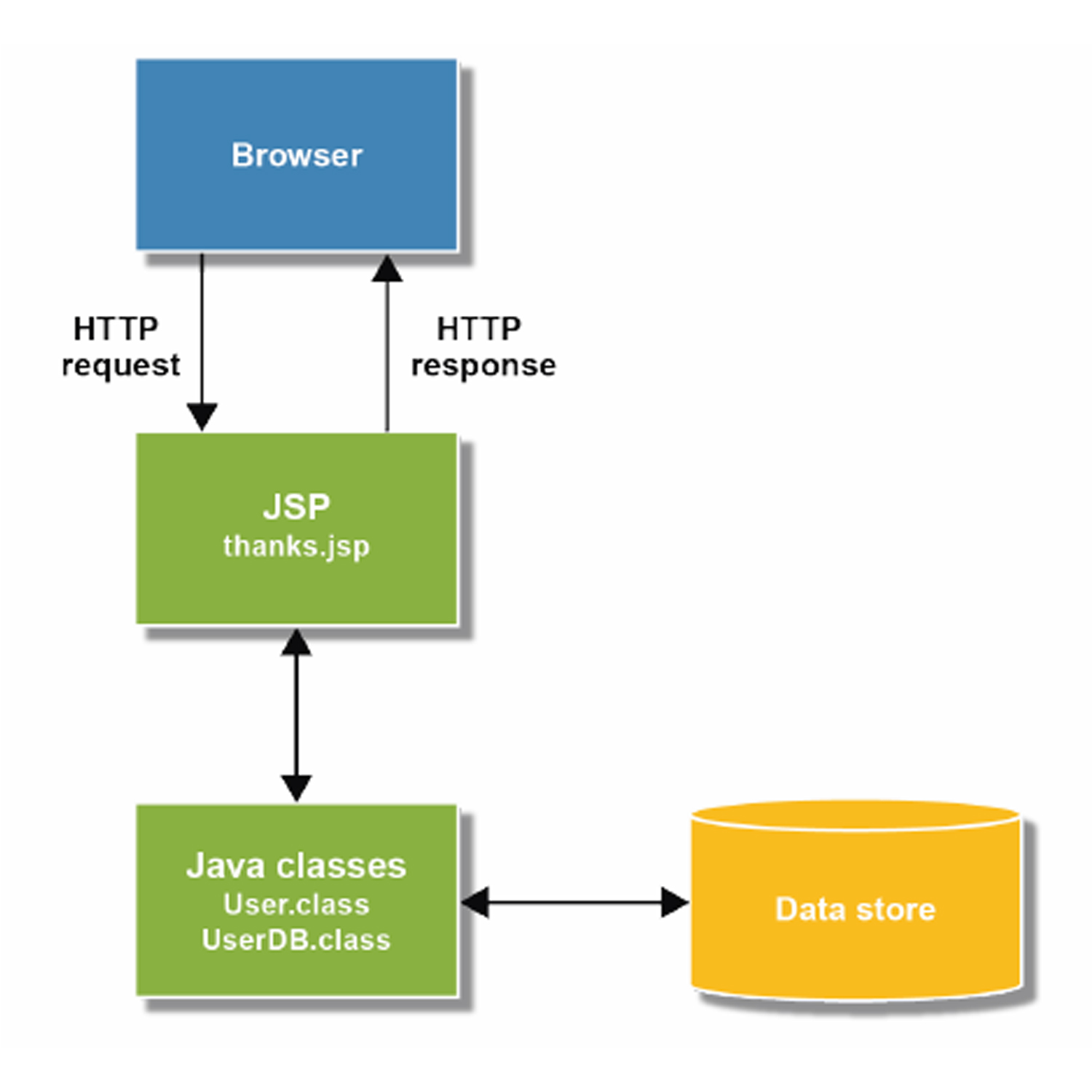
Description
- The Model 1 pattern uses JSPs to handle all of the processing and presentation for the application. This pattern is sometimes adequate for web applications with limited processing requirements, but it is generally considered a bad practice.
- In the Model 1 pattern, the JSPs can use regular Java classes to store the data of the application and to do the business processing of the application. In addition, they can use data classes to work with the data store.
- The data store is typically a database, but it can include disk files. This is often referred to as persistent data storage because it exists after the application ends.
The Model 2 (MVC) pattern
Figure 2-2 shows the Model 2 pattern. This pattern separates the code for the application into three layers: the model, the view, and the controller. As a result, this pattern is also known as the Model-View-Controller pattern (MVC pattern). This pattern works better than the Model 1 pattern whenever the processing requirements are substantial.
In the MVC pattern, the model defines the business layer of the application. This layer is usually implemented by JavaBeans, which you’ll learn more about in a moment. This type of class defines the data for the business objects and provides the methods that do the business processing.
The view defines the presentation layer of the application. Since it’s cumbersome to use a servlet to send HTML to a browser, an MVC application uses HTML documents or JSPs to present the view to the browser.
The controller manages the flow of the application, and this work is done by one or more servlets. To start, a servlet usually reads any parameters that are available from the request. Then, if necessary, the servlet updates the model and saves it to the data store. Finally, the servlet forwards the updated model to one of several possible JSPs for presentation.
Here again, most applications need to map the data in the model to a data store. But the JavaBeans usually don’t provide the methods for storing their own data. Instead, data access classes like the UserDB class provide those methods. That separates the business logic from the data access operations.
When you use the MVC pattern, you should try to keep the model, view, and controller as independent of each other as possible. That makes it easier to modify an application later on. If, for example, you decide to modify an application so it presents the view in a different way, you should be able to modify the view layer without making any changes to the controller or model layers. In practice, it’s difficult to separate these layers completely, but complete independence is the goal.
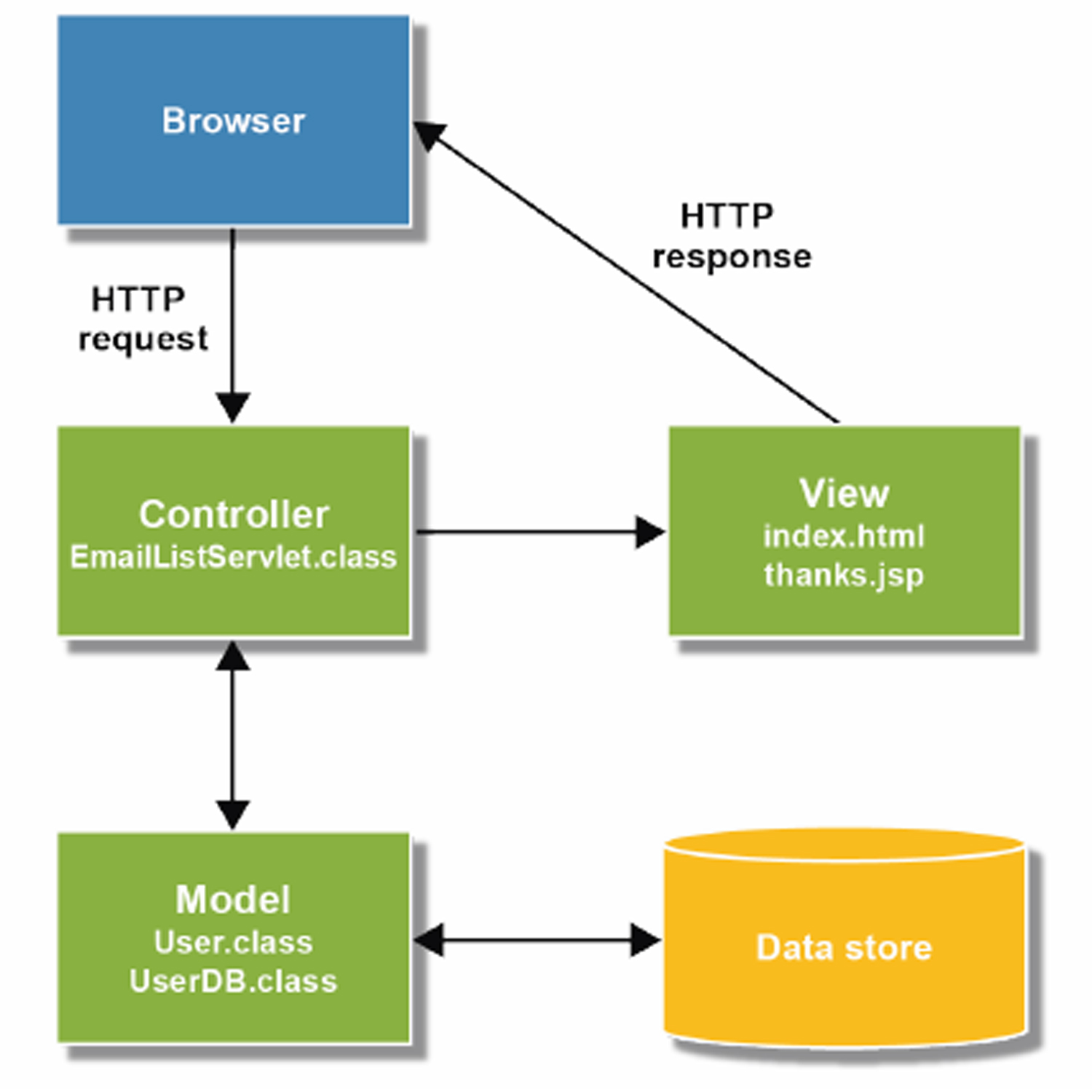
Description
- The Model 2 pattern separates the code into a model, a view, and a controller. As a result, it’s also known as the Model-View-Controller (MVC) pattern.
- The MVC pattern is commonly used to structure web applications that have significant processing requirements. That makes them easier to code and maintain.
- In the MVC pattern, the model consists of business objects like the User object, the view consists of HTML pages and JSPs, and the controller consists of servlets.
- Usually, the methods of data access classes like the UserDB class are used to read and write business objects like the User object to and from the data store.
- When you use the MVC pattern, you try to construct each layer so it’s as independent as possible. Then, if you need to make changes to one layer, any changes to the other layers are minimized.