Using PowerShell Operators
Like any other programming language, operators are a basic building block of PowerShell. When creating a script or module, chances are that you'll find that you need a PowerShell operator. There are several types of operators that can be used in PowerShell. For instance, there are operators for comparing values, operators for performing arithmetic, logical operators for comparing conditions, and, of course, operators for manipulating strings.
In this article, I'll go over the most common operators along with various examples of how they can be used. For further reading, take a look at the Microsoft help page too.
Assignment Operators
Variables are often used in PowerShell and they're created using the assignment operator. The simplest example of an assignment operator is the equal sign (=), which is used to assign a variable. Here, I assign a string to the variable $Testvar:
$Testvar = 'This is a string'
To add and remove items for a variable, use the add-equals (+=) and subtract-equals (?=) operators. In this example, I first assign 1 to $a, then add 2 and remove 1, which leaves the variable with a value of 2.
PS C:\> $a = 1
PS C:\> $a += 2
PS C:\> $a -= 1
PS C:\> $a
2
An interesting note is that if PowerShell contains only one object in a variable, the variable is automatically the data type of that object. This can be seen with the variable $a as the GetType method is used to display it is an integer:
PS C:\> $a.GetType()
IsPublic IsSerial Name
-------- -------- ----
True True Int32
To show the more standard arithmetic operators as well as how PowerShell gives precedence, consider this next example. First, the operation in the parentheses is processed (10-1) to equal 9. Next, 1 is multiplied by 5 and then 2 and 5 are added together. Finally, that sum (7) is added with 9 to give a result of 16.
PS C:\> 2+1*5+(10-1)16
The simplest example of an assignment operator is "=", which is used to assign a variable.
Comparison Operators
In PowerShell, comparison operators are commonly used to compare conditions for equality, matching, containment, and replacement. These operators, like the majority of other operators, are prefixed with a hyphen (-) such as -eq, which is used to verify if two values are equal.
To illustrate this, let's compare two variables against each other.
$a = 5
$b = 5
if ($a -eq $b){
"These are equal!"
}
else{
"These are not equal."
}
Because $a and $b are equal, the output will be These are equal!
In this next example, two date objects are compared with the greater than operator, which is -gt. The variable $Today gets the immediate date and time, and $Yesterday is used with the AddDays method to put the date and time to the day before. If you copy and paste this into the PowerShell console, the correct output is displayed as in Figure 1:

In PowerShell, comparison operators are very commonly used because they provide a technique for comparing conditions for equality, matching, containment, and replacement.
Table 1 shows additional examples of equality operators found in PowerShell.
Matching operators in PowerShell are used to compare strings against both wildcards and regular expressions to find a match. There are four operators in this category: -like, -notlike, -match, and -notmatch. In this next snippet, I create a foreach loop and go through each process running on my local system to see if any match “Drop*” using a wildcard.
foreach ($Process in Get-Process){
if ($Process.Name -like "Drop*"){
$Process.Name
}
}
Dropbox
Dropbox Web Hel
The output is a match for the Dropbox and Dropbox Web Helper processes.
For replacing part of a value in a string with another string the “-replace” operator is used.
Containment operators help find out whether a collection of objects contains a specific value, and if so, returns a Boolean after the first finding of the value. For example, -contains is used to find the process “powershell” in a list of processes. In this example the “powershell” process occurs twice in the list of processes; “True” is returned after finding the first instance.
PS C:\> Get-Process powershell |
Select-Object Name,Id
Name Id
---- --
powershell 7300
powershell 22744
PS C:\> (Get-Process).Name -contains
'powershell'
True
Similar to the -contains and -notcontains operators, PowerShell also provides -in and -notin, although these work pretty much the same. The only difference is the order in which they're used in relation to the collection and test value. With -notin, the test value must be used before the collection:
PS C:\> "powershell" -notin (Get-Process).Name
False
For replacing part of a value in a string with another string, the -replace operator is used. A simple example is the following snippet, which replaces the string this with that. Note the first parameter after -replace is the value to find and the second is the value to replace it with.
PS C:\> "Only replace this." -replace 'this.','that.'
Only replace that.
Logical Operators
In PowerShell, logical operators are used to connect and test multiple expressions and statements. Logical operators are excellent if testing is needed for complex expressions. Here, the output of Get-Process is piped to Where-Object and filtered with the -and operator to connect to find processes that start with Drop and don't start with Dropbox Web. The result is that Where-Object filters out the Dropbox Web Helper process but not Dropbox and DropboxUpdate, as shown in Figure 2.

Two other logical operators are -or and -not (which can be used as ! as well). In this if statement, the condition being tested is if either the “foo” or “powershell” process isn't found to be running with Get-Process, the output True is returned. Because the process “foo” isn't running (even though the “powershell” process is), the output is True.
PS C:\> Get-Process powershell | Select-Object -Property ProcessName
ProcessName
-----------
powershell
PS C:\> if (-not (Get-Process foo) -or !(Get-Process powershell)){
"True"
}
else {
"False"
}
True
Note that if the -or operator is changed to -and, keeping all the other if statement the same, the condition is false because both processes would need to be running for it to be true.
PS C:\> if (-not (Get-Process foo) -and !(Get-Process powershell)){
"True"
}
else {
"False"
}
False
One simple way to think of the difference between -or and -and is that -or means that just one aspect needs to be true in the statement, and -and means that all aspects of the conditions being tested need to be true.
When creating a script or module, chances are, you'll find that using a PowerShell operator is needed.
Split and Join Operators
To split and join strings to PowerShell, the -split and -join operators are used. These two operators are very commonly used in PowerShell scripts because this type of operation is necessary to manipulate strings. A simple example is taking a string that's formatted in a sentence and using -split to split the string at each blank space.
PS C:\> "This is a test sentence to split"
-split ' '
This
is
a
test
sentence
to
split
To best illustrate the -join operator, the previous string sentence can be placed into a variable and then concatenated to form the original string. Note the delimiter after the -join operator is " " indicating a space between strings.
PS C:\> $a = "This is a test sentence to split"
-split ' '
PS C:\> $a -join ' '
This is a test sentence to split
Another option for using -split is adding a script block after the operator. For instance, to split a string at either a comma or period, the -or operator can be used. In Figure 3, the string in $test is split in this exact way.
To split and join strings to PowerShell, the “-split” and “-join” operators are used.
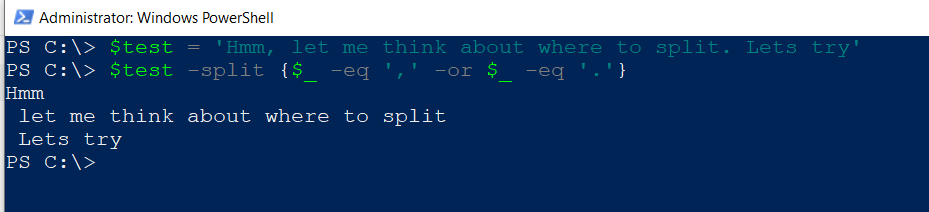
Summary
Much like the rest of the language, PowerShell operators are fairly easy to understand and use. Understanding the range of PowerShell operators and how they're used can help you build efficient and effective code. Operators offer ways to string together complex expressions in PowerShell.
Table 1: Other examples of equality operators in PowerShell
Operator | Description |
-ne | Not equal to |
-ge | Greater than or equal to |
-lt | Less than |
-le | Less than or equal to |