Five PowerShell cmdlets for Beginners
For years, Windows PowerShell has been the scripting language of choice for Microsoft IT professionals, and for good reason. The strength of PowerShell over other languages is the simplicity and readability that allows novice scripters and programmers learn it quickly. By default, in new Windows operating systems (currently Windows 10 and Windows Server 2019), installed right out of the box, there are thousands of cmdlets in PowerShell to use. In essence, these cmdlets can do just about anything on a Windows computer that might be done through a graphical user interface.
The sheer number can sometimes make it difficult for beginners to figure out where to start. Of course, there are cmdlets that are used quite frequently, not to mention cmdlets to make learning PowerShell easier.
PowerShell cmdlets are specialized classes within .NET.
PowerShell cmdlets (commands) are specialized classes within .NET. One of the more confusing aspects of PowerShell that users of other languages must understand is that in PowerShell, everything is an object and these objects have properties (properties are data about an object, such as a file name) and methods (operations that can be done to objects, such as deleting a file). You can't really use or think of PowerShell as the “Bash for Windows” because PowerShell doesn't work the same way as Bash. PowerShell structures much of the input data as objects automatically, unlike Bash, which uses text streams.
Get-Help
The Get-Help cmdlet is perhaps PowerShell's most valuable cmdlet as it allows a user to see what a specific cmdlet does, the parameters the cmdlet is used with, and best of all, gives examples of how the cmdlet is used. You can think of it as similar to the man
command on Linux. Even better, Get-Help displays information other than just the cmdlets, and you can learn about things such as operators and language concepts.
One of the more confusing aspects of PowerShell that users of other languages must understand is that in PowerShell, everything is an object.
In this first example, the -Name parameter is used to display help on the Add-Content cmdlet. In addition, the -Full parameter is used to show the entire help page on this topic, which includes a synopsis, parameter explanations, and examples.
PS C:\> Get-Help -Name Add-Content -Full
NAME
Add-Content
SYNOPSIS
Appends content, such as words or data, to a file.
For many developers, simply viewing examples of how a cmdlet is used is sufficient, so instead of specifying -Full, the -Examples parameter can be used as seen in Figure 1.
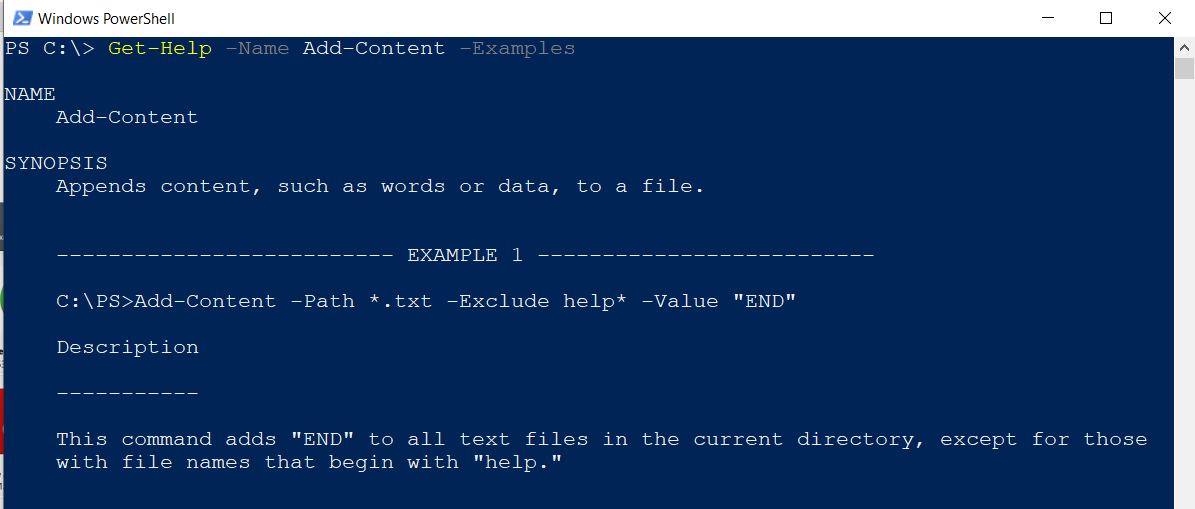
An often-overlooked aspect of Get-Help is the ability to view information on concepts in PowerShell. To view these topics in Get-Help, the concept is prefixed with about_
. For instance, to view information on the concept of comparison operators, the PowerShell command would be about_Comparison_Operators
.
PowerShell structures much of the data as input into objects automatically, unlike Bash.
One nice trick when you'd like to find a keyword in Get-Help is to use wildcards in the name parameter, which then returns the name of the topic.
PS C:\> (Get-Help -Name *operators*).Name
about_Arithmetic_Operators
about_Assignment_Operators
about_Comparison_Operators
about_Logical_Operators
about_Operators
about_Type_Operators
Get-Command
In PowerShell, a module is a group of cmdlets that are usually related in functionality, such as the ActiveDirectory module, which interacts with Active Directory. To view cmdlets from PowerShell modules installed on the local computer or in your current PowerShell session, the Get-Command is used. Similar to Get-Help, Get-Command isn't a cmdlet you'll likely use in a script, but it's very helpful when attempting to troubleshoot an issue while you're in a shell.
By default, simply running Get-Command displays every cmdlet installed locally in modules and snapins. This alone isn't much help, as the amount of output is significant.
A nice feature of Get-Command is the capability to find what cmdlets exist in a particular module. This is especially helpful when first learning what a module can do. To do this, the -Module parameter is used and the name of the module is stated, as can be seen below with the PackageManagement module.
PS C:\> Get-Command -Module PackageManagement
CommandType Name
----------- ----
Cmdlet Find-Package
Cmdlet Find-PackageProvider
Cmdlet Get-Package
Cmdlet Get-PackageProvider
Cmdlet Get-PackageSource
Cmdlet Import-PackageProvider
Cmdlet Install-Package
Cmdlet Install-PackageProvider
Cmdlet Register-PackageSource
Cmdlet Save-Package
Cmdlet Set-PackageSource
Cmdlet Uninstall-Package
Cmdlet Unregister-PackageSource
Get-ChildItem
The Get-ChildItem cmdlet is an alias for the dir
command in PowerShell (both in Windows PowerShell and PowerShell Core). Therefore, it lists items inside a folder if used on a file system, just like dir
. You may be thinking, “Why is this an important cmdlet?” It's a nice feature because it also works with PowerShell providers outside of a file system, including the Windows registry, environment variables, variables, and custom providers such as a VMware datastore.
In Figure 2, child items are shown inside the folder test
, which contains three text files. In the next command, output is piped to Where-Object and only items are selected that have the name text1.txt
. Note that Where-Object is a cmdlet used to filter objects in PowerShell based on certain values.
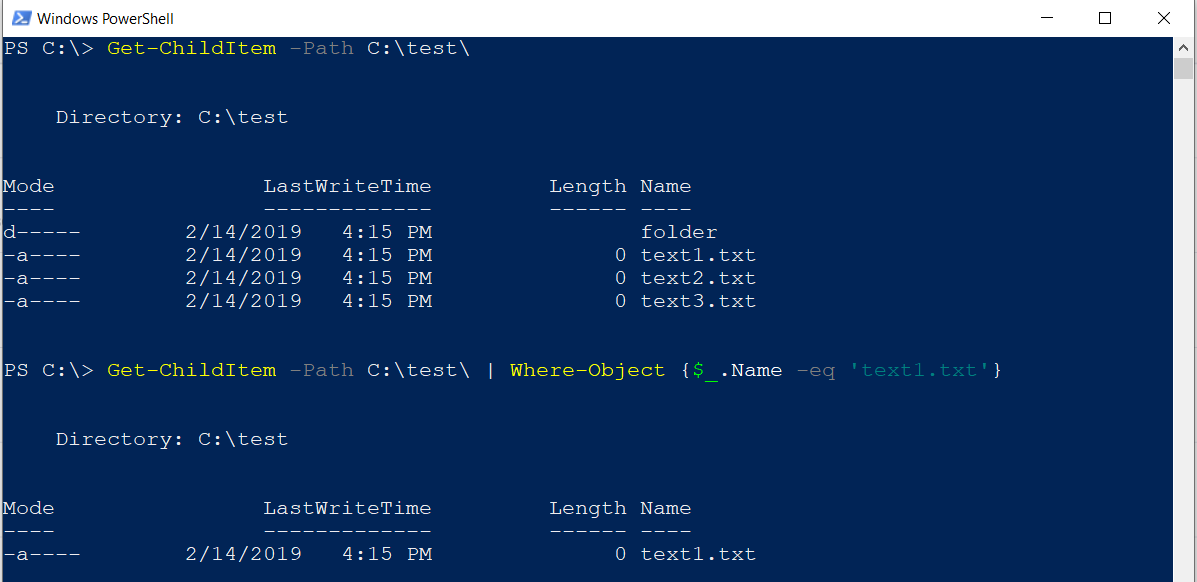
Get-ChildItem also works with PowerShell providers outside of a file system, including the Windows registry, environment variables, variables, and custom providers.
As mentioned previously, Get-ChildItem also interacts with the Windows registry. In this example, Get-ChildItem is used to display the registry key value of NoAutoUpdate
in Windows, which is a key that disables automatic Windows updates. Note that the pipe character ("|") can be used for multiline commands in the PowerShell console.
PS C:\> Get-ChildItem $RegKey |
>> Get-ItemProperty -Name NoAutoUpdate |
>> Select-Object -Property NoAutoUpdate
NoAutoUpdate
------------
0
Foreach-Object
Foreach loops are fundamental to any programming language, and PowerShell is no exception. This cmdlet simply performs a task on each object in a group of objects. Starting in version 3 of PowerShell, the Foreach-Object cmdlet is used to process objects with two different approaches. One approach is with a script block, using brackets. The other approach is simply calling a property or method directly.
In Figure 3, these two approaches are illustrated. In the first example, the -Process parameter is used to print the Name
property to the console. In the second example, the -Member parameter is used without a script block.

When used within a PowerShell script, the foreach
statement can be used without receiving input from the pipeline. Note that this is not the same as the Foreach-Object cmdlet and acts a bit differently. The foreach
statement requires a variable and collection that PowerShell will iterate. In the script block, the variable changes for each item that's included in the collection.
In this snippet, the variable is $item. Each time the $collection variable is iterated, the value of $item changes. In this case, first it's the string test1
and then it changes to test2.
$collection = 'test1','test'
foreach ($item in $collection){
Write-Output $Item
}
Get-Member
PowerShell is all about objects because pretty much everything in PowerShell is represented as an object. Although Get-Help and Get-Command show useful information about cmdlets and concepts in PowerShell, Get-Member displays information on the properties and methods on a given object.
In Figure 4, the folder test
is placed into a variable $test. Next, the Get-Member cmdlet runs to display the properties of this folder
object. This information is extremely useful because it provides an understanding of the properties a folder has that PowerShell can use.
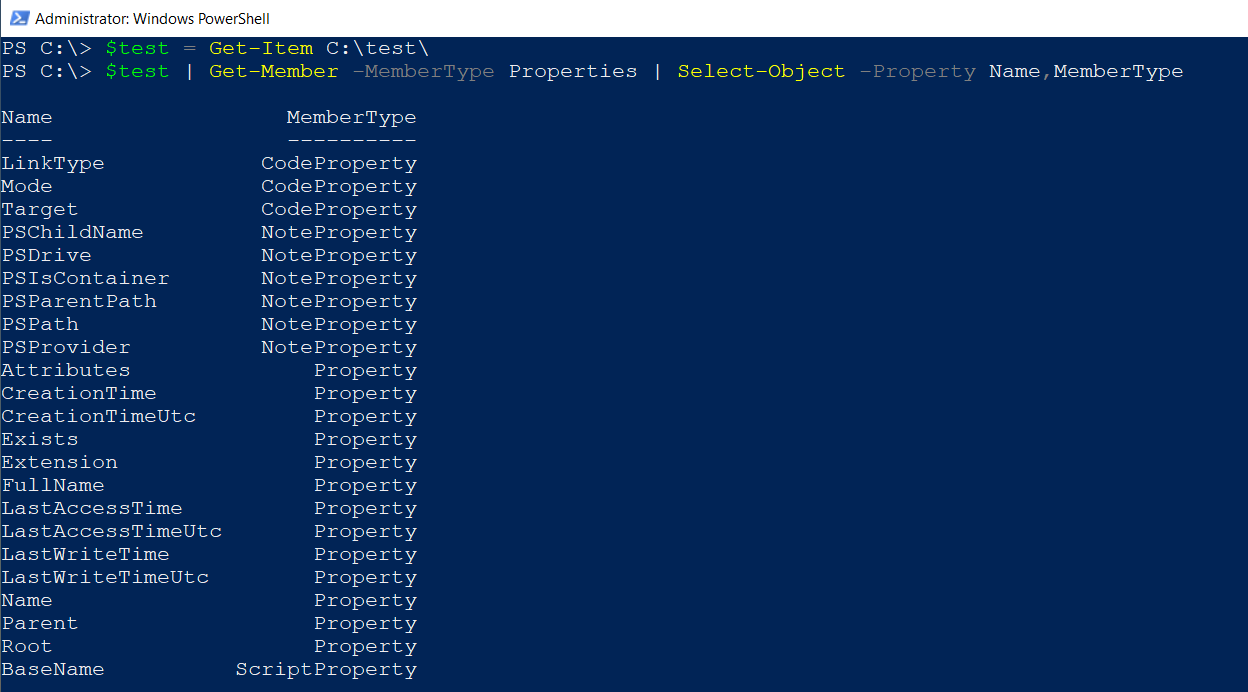
For instance, the FullName
property correlates to the full path to the folder and the CreationTime
property refers to the time the folder was created on the file system. To view the value of the CreationTime
property, $test variables are cycled through by pressing the tab key, which changes through methods and properties.
PS C:\> $test.CreationTime
Monday, October 17, 2016 3:23:39 PM
Summary
For IT professionals working with Windows, PowerShell is possibly the most useful tool to use for automating tasks. With the advent of PowerShell Core on Linux and Mac, these skills can be transferred to other operating systems as well, while using the same syntax. Even for users without any programming experience, PowerShell is very easy to learn and these cmdlets are a great place to start.