This excerpt is from the book, ‘Sams Teach Yourself ASP.NET 4 in 24 Hours: Complete Starter Kit, authored by Scott Mitchell, Published Jul 30, 2010 by Sams. Part of the Sams Teach Yourself-Hours series. ISBN 0-672-33305-8, Copyright 2011. For more info, please visit the publisher site http://www.informit.com/store/product.aspx?isbn=0672333058.
Scott Mitchell introduces you to ASP.NET, shows you how to install Visual Web Developer, along with the .NET Framework and SQL Server 2008, and helps you create your first ASP.NET page.
In this hour, we will cover
- What ASP.NET is
- The system requirements for using ASP.NET
- The software that must be installed prior to using ASP.NET
- Installing the .NET Framework, Visual Web Developer, and SQL Server 2008
- Taking a quick tour of Visual Web Developer
- Creating a simple ASP.NET page and viewing it through a web browser
ASP.NET is an exciting web programming technology pioneered by Microsoft that allows developers to create dynamic web pages. Dynamic web pages are pages whose content is dynamically regenerated each time the web page is requested. For example, after you sign in, the front page of Amazon.com shows recommended products based on your previous purchases. This is a dynamic web page because it is a single web page whose content is customized based on who is visiting. This book explores how to create such dynamic web pages using ASP.NET.
ASP.NET is a robust and mature technology. ASP.NET version 1.0 was released in January 2002 and quickly became the web programming technology of choice for many. In November 2005, Microsoft released the much-anticipated version 2.0. Two years later, in November 2007, Microsoft released ASP.NET version 3.5. And ASP.NET 4 was unveiled in April 2010.
Before we create our first ASP.NET website, we need to install the .NET Framework, Visual Web Developer, and SQL Server 2008. The .NET Framework is a rich platform for creating Windows-based applications and is the underlying technology used to create ASP.NET websites.
Visual Web Developer is a sophisticated program for creating, editing, and testing ASP.NET websites and web pages. ASP.NET web pages are simple text files, meaning that you can create them using any text editor (such as Microsoft Notepad), but if you've created websites before, you know that using a tool such as Microsoft Expression Web or Adobe Dreamweaver makes the development process much easier than using a generic text editor such as Notepad. This is the case for ASP.NET, as well.
SQL Server 2008 is a database engine, which is a specialized application designed to efficiently store and query data. Many websites interact with databases; any ecommerce website, for example, displays product information and records purchase orders in a database. Starting with Hour 13, "Introducing Databases," we'll see how to create, query, and modify databases from an ASP.NET page.
This hour focuses on installing the necessary software so that we can start creating ASP.NET web applications. We create a very simple ASP.NET page at the end of this hour, but we won't explore it in any detail. We look at ASP.NET pages in more detail in the next hour and in Hour 4, "Designing, Creating, and Testing ASP.NET Pages."
What Is ASP.NET?
Have you ever wondered how dynamic websites such as Amazon.com work behind the scenes? As a shopper at Amazon.com, you are shown a particular web page, but the web page's content is dynamic, based on your preferences and actions. For instance, if you have an account with Amazon.com, when you visit the home page your name is shown at the top and a list of personal recommendations is presented further down the page. When you type an author's name, a title, or a keyword into the search text box, a list of matching books appears. When you click a particular book's title, you are shown the book's details along with comments and ratings from other users. When you add the book to your shopping cart and check out, you are prompted for a credit card number, which is then billed.
Web pages whose content is determined dynamically based on user input or other information are called dynamic web pages. Any website's search engine page is an example of a dynamic web page because the content of the results page is based on the search criteria the user entered and the searchable documents on the web server. Another example is Amazon.com's personal recommendations. The books and products that Amazon.com suggests when you visit the home page are different from the books and products suggested for someone else. Specifically, the recommendations are determined by the products you have previously viewed and purchased.
The opposite of a dynamic web page is a static web page. Static web pages contain content that does not change based on who visits the page or other external factors. HTML pages, for example, are static web pages. Consider an HTML page on a website with the following markup:
<html>
<body>
<b>Hello, World!</b>
</body>
</html>
Such a page is considered a static web page because regardless of who views the page or what external factors might exist, the output will always be the same: the text Hello, World! displayed in a bold font. The only time the content of a static web page changes is when someone edits and saves the page, overwriting the old version.
By the Way
Virtually all websites today contain a mix of static and dynamic web pages. Rarely will you find a website that has just static web pages, because such pages are so limited in their functionality.
It is important to understand the differences between how a website serves static web pages versus dynamic web pages.
Did you Know?
ASP.NET is only one of many technologies that can be employed to create dynamic web pages. Other technologies include ASP-ASP.NET's predecessor-PHP, JSP, and ColdFusion. If you have experience developing web applications with other web programming technologies, you may already be well versed in the material presented in the next two sections. If this is the case, feel free to skip ahead to the "Installing the .NET Framework, Visual Web Developer, and SQL Server 2008" section.
Serving Static Web Pages
If you've developed websites before, you likely know that a website requires a web server.
A web server is a software application that continually waits for incoming web requests, which are requests for a particular URL (see Figure 1.1). The web server examines the requested URL, locates the appropriate file, and then sends this file back to the client that made the request.
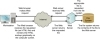
For example, when you visit Amazon.com, your browser makes a web request to Amazon.com's web server for a particular URL (say, /books/index.html). Amazon.com's web server determines what file corresponds to the requested URL and returns the contents of this file to your browser.
This model is adequate for serving static pages, whose contents do not change. However, such a simple model is insufficient for serving dynamic pages because the web server merely returns the contents of the requested URL to the browser that initiated the request. The contents of the requested URL are not modified in any way by the web server based on external inputs.
Serving Dynamic Web Pages
To accommodate dynamic content, dynamic web pages contain source code that is executed when the page is requested (see Figure 1.2). The executing code produces the HTML that is sent back to and displayed in the visitor's browser.

With this model, content isn't actually created until the web page is requested. Imagine that we wanted to create a web page that displays the current date and time. To do this using a static web page, someone would need to edit the web page every second, continually updating the content so that it contained the current date and time. Clearly, this isn't feasible.
With a dynamic web page, however, the executed code can retrieve and display the current date and time. Suppose that one particular user visits this dynamic page on August 1, 2010, at 4:15:03 p.m. When the web request arrives, the dynamic web page's code is executed, which obtains the current date and time and returns it to the requesting web browser. The visitor's browser displays the date and time the web page was executed: August 1, 2010, 4:15:03 p.m. If another visitor requests this page 7 seconds later, the dynamic web page's code will again be executed, returning August 1, 2008, 4:15:10 p.m.
Figure 1.2 is, in actuality, a slightly oversimplified model. Commonly, the web server and the software that executes the dynamic web page's source code are decoupled. When a web request arrives, the web server determines whether the requested page is a static web page or dynamic web page. If the requested web page is static, its contents are sent directly back to the browser that initiated the request (as shown in Figure 1.1). If, however, the requested web page is dynamic-for example, an ASP.NET page-the web server hands off the responsibility of executing the page to the ASP.NET engine (see Figure 1.3).
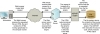
A common way that web servers determine whether the requested page is a dynamic or static web page is by the requested file's extension. For example, if the extension is .aspx, the web server knows the request is for an ASP.NET page and therefore hands off the request to the ASP.NET engine.
By the Way
The ASP.NET engine is a piece of software that knows how to execute ASP.NET pages. Other web programming technologies, such as ASP, PHP, and JSP, have their own engines, which know how to execute ASP, PHP, and JSP pages.
When the ASP.NET engine executes an ASP.NET page, the engine generates HTML output. This HTML output is then returned to the web server, which then returns it to the browser that initiated the web request.
Hosting ASP.NET Pages
To view an ASP.NET web page that resides on a web server, we need to request it using a web browser. The browser sends a request to the web server, which then dispatches the request to the ASP.NET engine. The ASP.NET engine processes the requested page, returns the resulting HTML to the web server, which then sends it back to the browser, where it is displayed to the user. When you're developing ASP.NET websites, the ASP.NET pages you create are saved on your personal computer. To test these pages, then, your computer must have a web server installed.
Fortunately, you do not need to concern yourself with installing a web server on your computer. Visual Web Developer, the editor we'll be using throughout this book to create our ASP.NET websites, includes a lightweight web server specifically designed for testing ASP.NET pages. As we will see in later hours, when testing an ASP.NET page, Visual Web Developer starts the ASP.NET Development Web Server and launches a browser that issues a request of the following form: http://localhost:portNumber/ASP.NET_Page.aspx.
The http://localhost portion of the request tells the browser to send the request to your personal computer's web server, in contrast to some other web server on the Internet. The portNumber specifies a particular port through which the request is made. All web servers listen for incoming requests on a particular port. When the ASP.NET Development Web Server is started, it chooses an open port, which is reflected in the portNumber portion of the URL. Finally, the ASP.NET_Page.aspx portion is the filename of the ASP.NET page being tested.
Hosting ASP.NET pages locally through the ASP.NET Development Web Server has a number of advantages:
- Testing can be done while offline-Because the request from your browser is being directed to your own personal computer, you don't need to be connected to the Internet to test your ASP.NET pages.
- It's fast-Local requests are, naturally, much quicker than requests that must travel over the Internet.
- Advanced debugging features are available-By developing locally, you can use advanced debugging techniques, such as halting the execution of an ASP.NET page and stepping through its code line-by-line.
- It's secure-The ASP.NET Development Web Server allows only local connections. With this lightweight web server, you don't need to worry about hackers gaining access to your system through an open website.
The main disadvantage of hosting ASP.NET pages locally is that they can be viewed only from your computer. That is, a visitor on another computer cannot enter some URL into her browser's Address bar that will take her to the ASP.NET website you've created on your local computer. If you want to create an ASP.NET website that can be visited by anyone with an Internet connection, you should consider using a web-hosting company.
Web-hosting companies have a number of Internet-accessible computers on which individuals or companies can host their websites. These computers contain web servers that are accessible from any other computer on the Internet. Hour 24, "Deploying Your Website," explores how to move an ASP.NET website from your personal computer to a web-hosting company's computers. After a website has been successfully deployed to a web-hosting company, you, or anyone else on the Internet, can visit the site.
By the Way
The examples and lessons presented in Hours 1 through 23 are meant to be created, tested, and debugged locally. Hour 24 contains step-by-step instructions for deploying your ASP.NET website to a web-hosting company.
Installing the .NET Framework, Visual Web Developer, and SQL Server 2008
Three components need to be installed before we can start building ASP.NET applications:
- The .NET Framework-Contains the ASP.NET engine, which is used to handle requests for ASP.NET pages. To install the .NET Framework engine, your computer must be running Windows XP, Windows Server 2003, Windows Vista, Windows Server 2008, or Windows 7.
- Visual Web Developer-The tool of choice for creating, editing, and testing ASP.NET pages.
- SQL Server 2008-A powerful database engine that is used extensively from Hour 14, "Accessing Data with the Data Source Web Controls," onward.
The book's CD includes the installation program for Visual Web Developer. Because Visual Web Developer is designed for developing ASP.NET websites, installing it automatically installs the .NET Framework and other required ASP.NET tools. You can also optionally install SQL Server 2008.
To begin the installation process, insert the CD into your computer. This brings up the installation program starting with the screen shown in Figure 1.4.
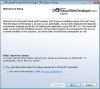
Click the Next button twice to advance past the welcome and license terms screens. At this point you should see the screen shown in Figure 1.5. From here, you can choose whether to install Microsoft SQL Server 2008 Express Edition. Installing SQL Server 2008 is optional in the sense that Visual Web Developer will install successfully with or without it; however, the latter half of this book's examples rely on SQL Server 2008 being installed. Therefore, make sure that this check box is selected.

By the Way
The "Installation Options" screen shown in Figure 1.5 is only displayed if you do not have SQL Server 2008 Express Edition already installed on your computer. If you already have this software installed, you will be taken directly to the step shown in Figure 1.6.
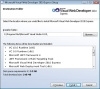
The next screen (see Figure 1.6) enables you to specify in what folder to install Visual Web Developer as well as what products will be installed and the disk space required.
After double-checking that the correct packages are being installed, click the Install button to begin the installation process. The overall installation process will take several minutes. During the installation, you are kept abreast with what package is currently being installed as well as the overall installation progress (see Figure 1.7).

A Brief Tour of Visual Web Developer
When the installation process completes, take a moment to give Visual Web Developer a test spin. To launch Visual Web Developer, go to the Start menu, choose Programs, and click Microsoft Visual Web Developer 2010 Express. Figure 1.8 shows Visual Web Developer after it has loaded.
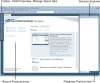
When you open Visual Web Developer, the Start Page is initially shown. This Start Page includes a list of recent projects on the left and a Get Started section on the right that includes links for accomplishing common tasks. There's also a Latest News tab on the Start Page that lists recent developer articles from Microsoft's website.
On the far left you'll find tabs for the Toolbox, CSS Properties, and Manage Styles windows. You can expand these windows by hovering your mouse over the tabs. At this point, these three windows are empty, but when you're creating or editing an ASP.NET page they'll include a variety of options. For example, the Toolbox is where you'll find the plethora of ASP.NET Web controls that can be added to an ASP.NET page. (We'll discuss what Web controls are and their purpose in the next hour.) The CSS Properties and Manage Styles windows are used to define style and appearance settings for the HTML and Web control elements within a web page.
On the right of the screen, you'll find the Solution Explorer. From the Start Page the Solution Explorer is empty, but when you load or create an ASP.NET website, the Solution Explorer will list the website's files. These files include database files, HTML pages, ASP.NET pages, image files, CSS files, configuration files, and so on. In addition to the Solution Explorer, the right portion of the screen is also home to the Database Explorer. The Database Explorer lists the databases associated with the project and provides functionality for creating, editing, and deleting the structure and contents of these databases.
Creating a New ASP.NET Website
To create and design an ASP.NET page, we must first create an ASP.NET website. There are several ways to create a new ASP.NET website from Visual Web Developer. You can go to the File menu and choose the New Web Site option; you can click the New Website icon in the toolbar; or you can click the New Web Site link above the Recent Projects pane of the Start Page.
All these approaches bring up the New Web Site dialog box, which is shown in Figure 1.9. Let's take a moment to create a website. For now, don't worry about all the options available or what they mean because we'll discuss them in detail in Hour 3, "Using Visual Web Developer." By default, the New Web Site dialog box should have Visual Basic as the selected programming language, ASP.NET Web Site as the selected website template, and the File System option selected in the Web location drop-down list. Change the website template from ASP.NET Web Site to Empty Web Site. Next, change the location of the website so that it will be created in a folder named MyFirstWebsite on your desktop. You can type in the name or click the Browse button to select the folder.

After you create the new website, your screen should look similar to Figure 1.10.
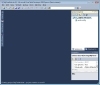
Watch Out!
When you are creating a new website using the Empty Web Site template, Visual Web Developer adds a single configuration file to the project named web.config. Using other templates causes Visual Web Developer to add many more files and folders to the new website. For example, if you selected the ASP.NET Web Site template, your new website would have folders named Account, App_Data, Scripts, and Styles, along with files such as About.aspx, Default.aspx, Global.asax, and others. These added files can be helpful if you are already familiar with ASP.NET, but can be distracting when learning about ASP.NET.
If you accidentally selected a template other than the Empty Web Site template, go to the File menu, choose New Web Site, and create a new website using the correct template.
Creating a Simple ASP.NET Page
When you fire up your browser and visit a website, your browser requests a particular web page from the web server. At the moment, our website does not yet contain any ASP.NET pages, which means there is no way to visit the site. Let's add an ASP.NET page to our website.
Right-click the website name in the Solution Explorer and choose the Add New Item menu option. This brings up the Add New Item dialog box shown in Figure 1.11. The default settings add a new ASP.NET page to the project named Default.aspx. We'll examine this dialog box and its various options in Hour 3. For now, make sure that the Visual Basic programming language and Web Form item templates are selected. Ensure that the name is set to Default.aspx and that the Place code in separate file check box is checked, and then click the Add button.
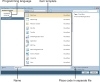
Figure 1.12 shows Visual Web Developer after the Default.aspx page has been added to the website. Note that Default.aspx is listed in the Solution Explorer and that its contents are displayed in the main window.

Right now Default.aspx consists of just HTML. As we will see in future hours, ASP.NET pages can also contain Web controls and server-side source code. Typically, ASP.NET pages are broken into two files: one that contains the HTML markup and Web control syntax, and another that contains the source code. If you expand Default.aspx in the Solution Explorer, you'll see that there's another file, Default.aspx.vb. This is the source code file for Default.aspx.
By the Way
Don't worry if you're feeling a bit overwhelmed. The point of this hour is to give a cursory overview of Visual Web Developer. Over the next three hours, we'll look at the portions of an ASP.NET page and the steps involved in creating and testing ASP.NET pages in much greater detail.
There are three "views" from which you can work with the HTML elements and Web controls in an ASP.NET page. The first is the Source view, which shows the page's HTML markup and Web control syntax. This is the default view and the one shown in Figure 1.12. The second view, called the Design view, provides a simpler alternative to specifying and viewing the page's content. In the Design view you can drag and drop HTML elements and Web controls from the Toolbox onto the design surface. You don't need to type in the specific HTML or Web control syntax. The third view, Split, divides the screen in half, showing the Source view in the top portion and the corresponding Design view in the bottom. You can toggle between the Source, Design, and Split views for an ASP.NET page by using the Design, Source, and Split buttons at the bottom of the main window or by typing Shift-F7 on your keyboard.
Did you Know?
You can reposition the Properties, Toolbox, Solution Explorer, Database Explorer, and other windows by clicking their title bars and dragging them elsewhere, or you can resize them by clicking their borders. If you accidentally close one of these windows by clicking the X in the title bar, you can redisplay it from the View menu.
Testing the ASP.NET Page
For us to view or test an ASP.NET page, a browser needs to make a request to the web server for that web page. Let's test Default.aspx. Before we do, though, let's add some content to the page, because right now the page's HTML will not display anything when viewed through a browser. From the Source view, place your cursor between the <div> and </div> tags in Default.aspx and add the following text:
<h1>Hello, World!</h1>
This displays the text Hello, World! in a large font. After entering this text, go to the Solution Explorer, right-click Default.aspx, and choose the View in Browser menu option. This starts the ASP.NET Development Web Server and launches your computer's default browser, directing it tohttp://localhost:portNumber/MyFirstWebsite/Default.aspx (see Figure 1.13). The portNumber portion in the URL will depend on the port selected by the ASP.NET Development Web Server.
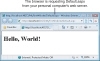
This ASP.NET page isn't very interesting because its content is static. It does, however, illustrate that to view the contents of an ASP.NET page, you must start the ASP.NET Development Web Server and request the page through a browser.
Summary
Today, virtually all websites contain dynamic web pages of one kind or another. Any website that allows a user to search its content, order products, or customize the site's content is dynamic in nature. A number of competing technologies exist for creating dynamic pages-one of the best is ASP.NET.
Throughout this book we'll be examining how to create interactive and interesting ASP.NET pages. You will implement the examples using Visual Web Developer, a free editor from Microsoft designed specifically for working on ASP.NET websites. In the "Installing the .NET Framework, Visual Web Developer, and SQL Server 2008" section, we looked at how to install Visual Web Developer, along with the .NET Framework and SQL Server 2008. In the "A Brief Tour of Visual Web Developer" section, we poked around Visual Web Developer and created our first ASP.NET page, testing it through a browser. In Hour 3, we'll explore the Visual Web Developer environment in much greater detail.
We have just begun our foray into the world of ASP.NET. Over the next 23 hours, we'll explore the ins and outs of this exciting technology!
Q&A
- Q. What is the main difference between a static and a dynamic web page?
- A. A static web page has content that remains unchanged between web requests, whereas a dynamic web page's content is generated each time the page is requested. The dynamic content is typically generated from user input, database data, or some combination of the two.
- For an example of a dynamic web page, consider the official website for the National Basketball Association (www.NBA.com), which lists team schedules, ongoing game scores, player statistics, and so on. This site's web pages display information stored in a database. Another example is a search engine site such as Google, which displays dynamic content based on both its catalog of indexed websites and the visitor's search terms.
- Q. You mentioned that with Visual Web Developer we'll be using the ASP.NET Development Web Server. Are other web server systems available for serving ASP.NET pages?
- A. The ASP.NET Development Web Server is designed specifically for testing ASP.NET pages locally. The computers at web-hosting companies use Microsoft's Internet Information Server (IIS), which is a professional-grade web server designed to work with Microsoft's dynamic web technologies-ASP and ASP.NET.
- If you are running Windows XP Professional, Windows Server 2003, Windows Vista, Windows 7, or Windows Server 2008, IIS may already be installed on your computer. If not, you can install it by going to Start, Settings, Control Panel, Add or Remove Programs, and clicking the Add/Remove Windows Components option.
- I encourage you not to install IIS and to instead use the ASP.NET Development Web Server unless you are already familiar with IIS and know how to administer and secure it. The ASP.NET Development Web Server is more secure because it allows only incoming web requests from the local computer; IIS, on the other hand, is a full-blown web server and, unless properly patched and administered, can be an attack vector for malicious hackers.
Workshop
Quiz
- What is the difference between a static web page and a dynamic web page?
- What is the purpose of the ASP.NET engine?
- True or False: ASP.NET pages can include Visual Basic or C# source code.
- What software packages must be installed to serve ASP.NET pages from a computer?
- When should you consider using a web-hosting company to host your ASP.NET website?
Answers
- The HTML markup for a static web page remains constant until a developer actually modifies the page's HTML. The HTML for a dynamic web page, on the other hand, is produced every time the web page is requested.
- When the web server receives a request for an ASP.NET page, it hands it off to the ASP.NET engine, which then executes the requested ASP.NET page and returns the rendered HTML to the web server. The ASP.NET engine allows for the HTML of an ASP.NET page to be dynamically generated for each request.
- True.
- For a computer to serve ASP.NET pages, the .NET Framework and a web server that supports ASP.NET must be installed.
- You should consider using a web-hosting company if you want your ASP.NET website to be accessible via the Internet. Typically, it's best to first develop the website locally and then deploy it to a web-hosting company when you are ready to go live.
Exercises
This hour does not have any exercises. We'll start with exercises in future hours, after we become more fluent with creating ASP.NET pages.
© 2012 Pearson Education, Inc. All rights reserved. 800 East 96th Street Indianapolis, Indiana 46240