Finding what you need in the Microsoft® Visual Studio® .NET documentation, which has over 45,000 topics, can be a daunting task. The Doc Detective is here to help, utilizing his investigative skills to probe the depths of the documentation.
Can't find what you're looking for? Just ask - if it's in there, I'll find it for you; if it isn't, I'll let you know that as well (and tell you where else you might go to find it).
Have a question for the Doc? Send your questions for future columns to me at docdetec@microsoft.com.
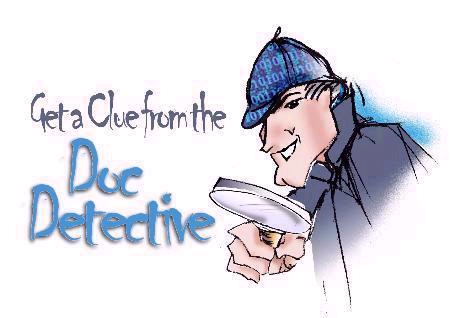
Dear Doc Detective,
In earlier versions of Visual Basic you could use the ZOrder method to set the layering of controls, that is, to determine which control appeared over the top of another. I've searched high and low, but I can't find a ZOrder method or property in the .NET. Surely there must be some way to do this.
- Zapped in Zanesville
Dear Zapped,
There is no reason to lose sleep over this; you can indeed still control the ZOrder using the .NET Framework. The designers of the framework chose to replace the ZOrder method with two methods: BringToFront() and SendToBack().
In Visual Basic 6 you would have used the following to bring a label to the front of the ZOrder.
Label1.ZOrder = vbBringToFront
In Visual Basic.NET, the same code would look like this.
Label1.BringToFront()
There are a number of seemingly arbitrary differences between Visual Basic 6 and Visual Basic .NET, but there are a whole series of topics that map VB 6 properties, methods and events to their .NET equivalents. Just look under "Introduction to Visual Basic .NET for Visual Basic Veterans" in the table of contents and drill down from there.
All this talk about ZOrder is making the Doc sleepy ? think I'll go catch some Z's.
- Doc D
Dear Doc Detective,
I like this new Console project type that's available in Visual Studio .NET. I'm working on a utility program that parses text files. I want to replace my text prompts with command line switches. How do I program that, and how do I send the switches to the program when I press F5? Here's an example of my command line.
parsefile filename.txt fast verbose.
Please help!
--Inconsolable in Independence
Dear Inconsolable,
You have several options for grabbing that extra text; I'll show you two. If you don't expect any of the arguments to be enclosed in quotes (like "My Documents"), then I suggest the Command function that is part of the Visual Basic runtime. That command puts everything that follows the program name into a string. You can use the String.Split function to break out the individual words into an array of strings. Your Main function would look like this.
Sub Main()
Dim switchLine As String = Command()
Dim switches() As String =
switchLine.Split(" ")
' Now use the switches
End Sub
Unfortunately, the method above would break "My Documents" into two strings, "My" and "Documents". To prevent this, you can use the .NET Framework System.Environment.GetCommandLineArgs method to retrieve the line. The following method also retrieves the program name.
Sub Main()
Dim switches() As String
switches =
System.Environment.GetCommandLineArgs()
' Now use the switches
End Sub
To specify the arguments used when you press F5, open the Project Properties dialog box by right-clicking the project in Solution Explorer and selecting Properties. Select the Debugging page of the Configuration Properties, and then set the Command line arguments property.
For a discussion of these and other command line options, look for the topics "The Visual Basic Version of Hello World!" and "Structure of a Visual Basic Program." Hopefully this will put you back in command.
- Doctor D
Dear Doc Detective,
My boss is asking me to create a search application for him. He wants to be able to click an icon on the desktop, enter the text to search for, and automatically launch a Web page with the search results. How would I write a script to do this?
- Confused in Conshohocken
Dear Confused,
If I understand you correctly, you don't actually need a script, just a simple Windows Forms application. I don't know of anything in the documentation that describes this, but here's an example of launching a search using the MSN search engine:
Create a simple form with a button and a textbox, and add the following line of code to the button's Click event.
Process.Start("http://search.msn.com/results.as
p?FORM=sCPN&RS=CHECKED&un=doc&v=1&q=" &
TextBox1.Text)
When you run this application, enter the term to search for in the textbox, then click the button - it will launch your browser and open the search results page.
To install the application as a desktop icon, see the topic "Adding and Removing Shortcuts in the File System Editor" in the MSDN Library.
It also sounds like your boss might already have a favorite search engine. If so, just replace the MSN search URL with the URL for that search engine. You may need to run a search first to get the exact format for the URL.
- the Doc
Dear Doc Detective,
I'm working with the Windows DataGrid control in VB.NET. I'm using the code
DataGrid1.Item(DataGrid1.CurrentRowIndex, 2)
to get a column value from the selected row. This works fine when the user clicks the grid if the code is in the DataGrid1_MouseUp event. But when the user clicks a column header to sort the grid, the value that is returned is the old value (before the grid is updated after sort). Can I place the code in another event, or is there an event that tells me when the sorting is finished?
- Gridlocked in Grand Rapids
Dear Gridlocked,
As you've discovered, the DataGrid control poses some unique challenges. When capturing mouse events, you need to know where the user clicked - on a cell, on a row header, on a column header, and so forth. Fortunately the DataGrid provides a HitTest method that returns a HitTestType enumeration that tells you where the user clicked. In your case, you need to call the HitTest method and if it returns HitTestType.ColumnHeader, you ignore the value since the current cell hasn't changed.
Look for the topic "Responding to Clicks in the Windows Forms DataGrid Control" in the MSDN Library for an example of the HitTest method.
By the way, unless you specifically want to capture only mouse events, you might take a look at the CurrentCellChanged event. Using this event allows you to retrieve the value of the cell when a user navigates the DataGrid via the keyboard as well. The aforementioned topic also includes an example of the CurrentCellChanged event.
- Doc Detective
Doc's Doc Tip(s) of the Day
When you get a list of search results, the items are "ranked" by how many times the search terms occur in each article. But you can easily rearrange the list to find the items most relevant to you. The Location column of the results list names the product or feature area that an article belongs to. Click the Location column heading to alphabetically sort your result list by Location name. Once you've done this you can easily see the group of articles related to the product, language, or technology that is most relevant to you. Likewise, you can click the Title column heading to alphabetically sort the results by article title. This can be helpful if you remember the name of an article you are looking for but are having trouble picking it out of a long list of articles.
Found a topic in Help that doesn't help? Tell the Visual Studio documentation team about it at vsdocs@microsoft.com.
URLs
http://msdn.microsoft.com/library/default.asp?url=/library/en-us/vbcn7/html/vbconhelloworld.asp